Building Minimal Docker Image for Go Applications
Learn how to build tiny docker image for Go from scratch
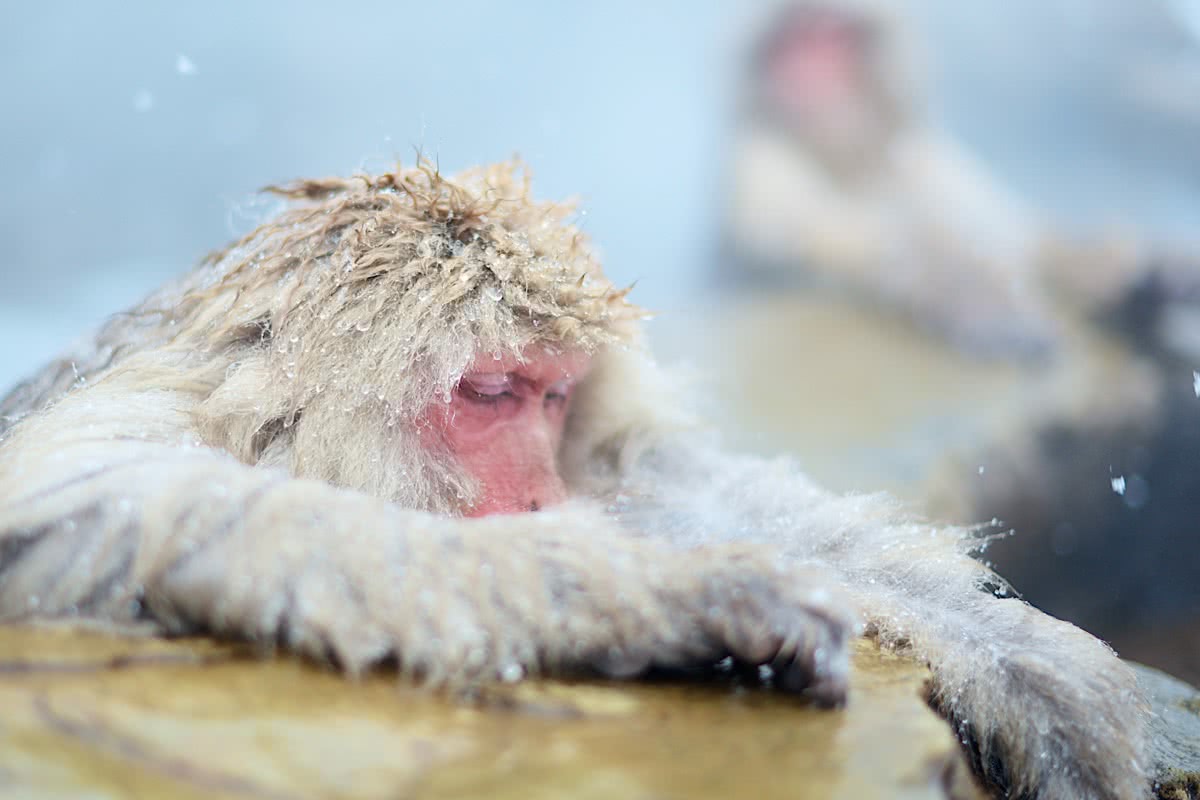
Table of Contents
Lets create a simple small application that keeps printing out Hello World!
// main.go
package main
import (
"fmt"
"time"
)
func main() {
for {
fmt.Println("Hello World!")
time.Sleep(1 * time.Second)
}
}
CGO_ENABLED
let the Go binaries link the libraries on the system. To reduce the binary size, CGO_ENABLED is enabled by default for native build. This time we use scratch
as our base image, which is a special Docker image with nothing in it (even the libraries), we need to disable the cgo parameter let compiler packages all the libraries application need into the binary.
If you want to do cross compilation
, dont forget to add GOOS
and GOARCH
.
$ CGO_ENABLED=0 GOOS=linux GOARCH=amd64 go build -o main
Here is all we need in the Dockerfile
FROM scratch
ADD main /
CMD ["/main"]
$ docker build -t minimal_go_docker_img .
$ docker images
REPOSITORY TAG IMAGE ID CREATED SIZE
minimal_go_docker_img 1.0 8f836c8b219a 17 minutes ago 1.638 MB
$ docker run --rm minimal_go_docker_img
If your application need to access https service, dont forget to add the ca.crt.
Normally you can find ca-certificates.crt
under the /etc/ssl/certs/
at any linux distribution.
FROM scratch
ADD ca-certificates.crt /etc/ssl/certs/
ADD main /
CMD ["/main"]
See Also
- Kubernetes - Two Steps Installation
- Adopting Container and Kubernetes in Production
- Rolling Updates with Kubernetes Deployments
- Kubernetes - Assigning Pod to Nodes
- Kubernetes - Pod
To reproduce, republish or re-use the content,
please attach with link: https://tachingchen.com/
Twitter
Google+
Facebook
Reddit
LinkedIn
StumbleUpon
Pinterest
Email