Pitfall of Go HTTP Header Operation
Use example to explain common mistakes when operating HTTP Header in Go
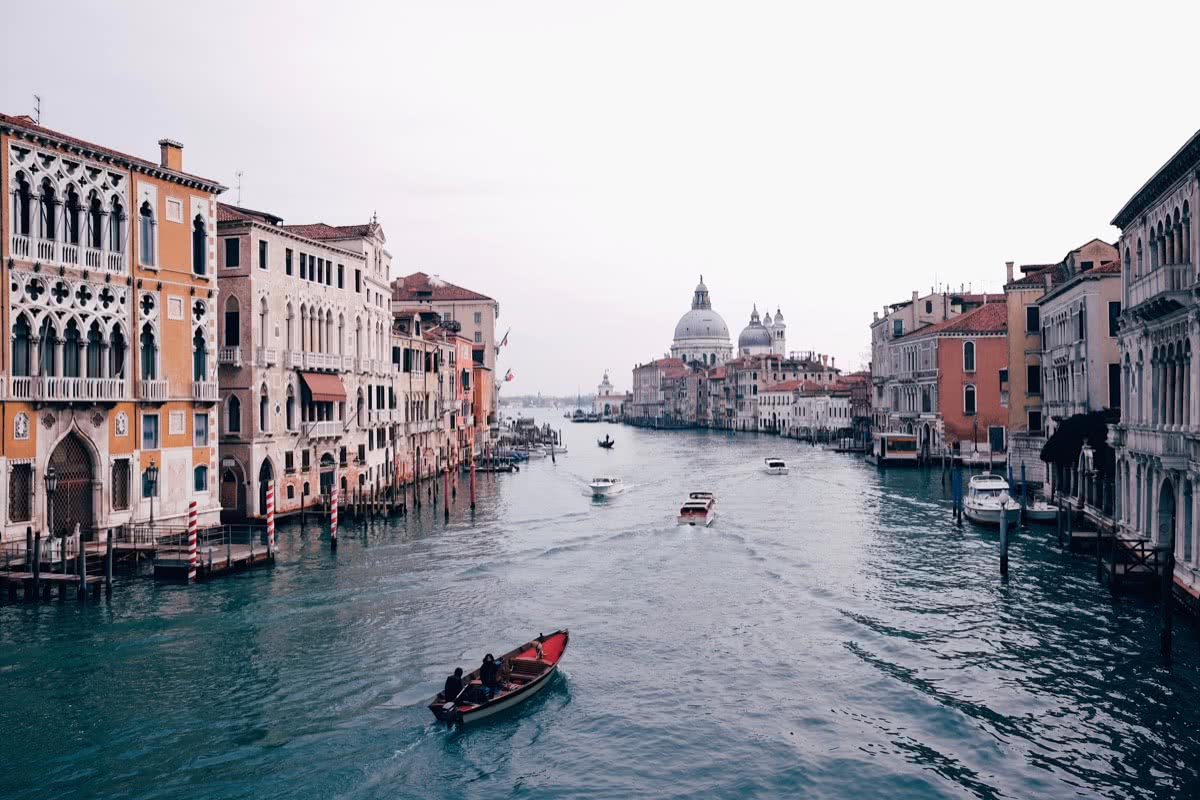
Preface
Most of the recent works are working on manipulating HTTP header in Go and noticed some common mistakes people may fall into while in development. So following we will go through some examples to see how to prevent and resolve these problems.
All sample code can be found at Github Repo。
ResponseWriter.Write causes client receives wrong HTTP status code
First, let’s see the following function and guess what HTTP status code user will receive.
http.HandleFunc("/", func (w http.ResponseWriter, r *http.Request) {
w.Write([]byte("foobar"))
w.WriteHeader(http.StatusBadRequest)
})
400 Bad Request? No, the answer is 200 OK。
$ curl -i http://127.0.0.1:8080
HTTP/1.1 200 OK
Date: Thu, 20 Dec 2018 06:31:33 GMT
Content-Length: 6
Content-Type: text/plain; charset=utf-8
foobar